Notice. Article updated in February 2018 for latest Laravel 5.6 version.
If you follow proper development and deployment process, at some point you have to test your web app with some kind of data. Fake data, preferably. But, on the other hand, "real enough" to test how it would look and work. There's an awesome package for this called Faker.
It's not exactly Laravel package, but perfectly works with this framework as well. As of today, its latest version is 1.7, released in August 2017.
So, what can Faker do? Basically, fake some data for you. Usually, in Laravel case, it is used within the Seeds. So let's see real life situation.
Let's take an example from the official Laravel documentation on Seeding. As the framework comes with users table migration, we will use it for our example. Here's how seed file looks:
use Illuminate\Database\Seeder; use Illuminate\Support\Facades\DB; class DatabaseSeeder extends Seeder { /** * Run the database seeds. * * @return void */ public function run() { DB::table('users')->insert([ 'name' => str_random(10), 'email' => str_random(10).'@gmail.com', 'password' => bcrypt('secret'), ]); } }Basically, this example does exactly what we need - fakes a name and an email of a user. With Faker, we can do much more than this.
How (NOT) to install Faker
If you wonder how to install it, good news - it's already installed for you in Laravel! Take a look at a default composer.json of Laravel:"require-dev": { "filp/whoops": "~2.0", "fzaninotto/faker": "~1.4", "mockery/mockery": "~1.0", "nunomaduro/collision": "~2.0", "phpunit/phpunit": "~7.0", "symfony/thanks": "^1.0" },The second line of this list is exactly what we need. The version is not the latest, but that doesn't make too much difference - most of the functions are still available. So, we can start using Faker straight away.
Seeding fake data
We will rewrite our example, using Faker and generating 10 users instead of 1:use Illuminate\Database\Seeder; use Illuminate\Support\Facades\DB; use Faker\Factory as Faker; class DatabaseSeeder extends Seeder { /** * Run the database seeds. * * @return void */ public function run() { $faker = Faker::create(); foreach (range(1,10) as $index) { DB::table('users')->insert([ 'name' => $faker->name, 'email' => $faker->email, 'password' => bcrypt('secret'), ]); } } }That's it - $faker->name will generate a random person name, and $faker->email - a random email. After we run artisan db:seed, results would look something like this:
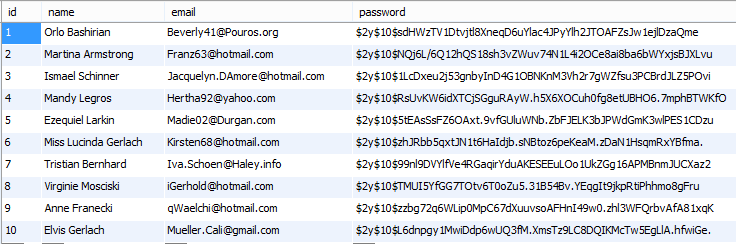
Want more articles like this every week? Subscribe!
What else can Faker generate?
A lot, actually. You would be amazed. There's a huge list of its functions - the author calls them Formatters. By the way, official documentation of Faker is awesome. Here are only a few of widely used examples:$faker->randomDigit; $faker->numberBetween(1,100); $faker->word; $faker->paragraph; $faker->lastName; $faker->city; $faker->year; $faker->domainName; $faker->creditCardNumber;And there's a lot lot more - refer to the official list. Some of those formatters have additional parameters for customization.
But it's only in English, right?
No. That's the whole point - the whole system of "faking" is based on Locales. By default, you work with en_US texts, but you can add your own providers and logic in your language - a list of supported languages is already huge. So, by choosing different locale, you can generate, for example:$faker->region; // fr_FR Region "Saint-Pierre-et-Miquelon" $faker->bankAccountNumber; // pl_PL "PL14968907563953822118075816" $faker->cellNumber; // nz_NZ "021 123 4567"So, actual Faker is just a core functionality, which can be expanded by your needs. Why wait? Try Faker for your next project! What other useful packages do you know to work with testing or fake data?
No comments or questions yet...