Quite often, especially in e-shop projects, you can see a page to login or register before finishing the purchase. But Laravel has these two routes as separate login and register pages. So how to merge them together and avoid conflicts?
Notice: at the end of this article, you will find a link to Github project with a simple Laravel checkout process.
This is the pre-checkout page we're aiming for:
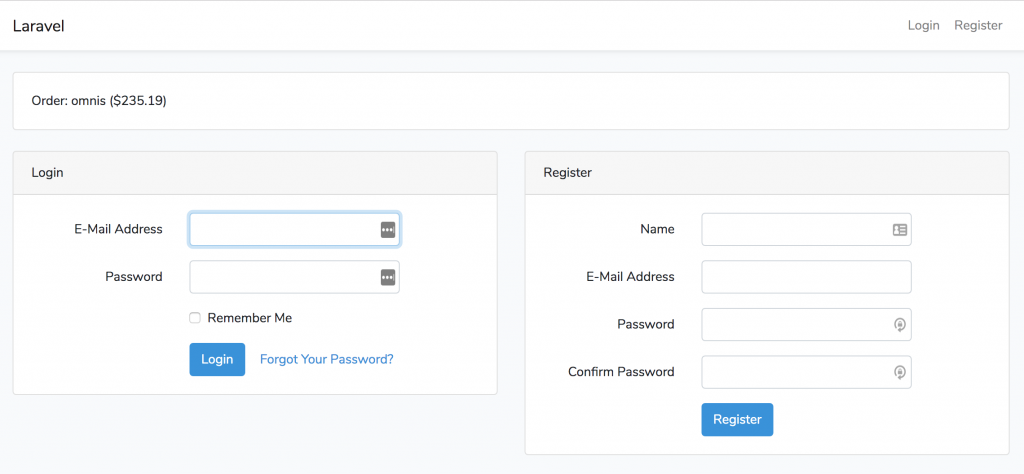
On the surface, it's pretty easy - just copy/paste all the Blade code from /resources/views/auth/login.blade.php and /resources/views/auth/register.blade.php into one common, let's say, /resources/views/checkout.blade.php. And that's true, but that's only one part of the story.
The problem arises because of the same names of fields.
Login form has this:
<input id="email" type="email"
class="form-control @error('email') is-invalid @enderror" name="email"
value="{{ old('email') }}" required autocomplete="email" autofocus>
Register form has this:
<input id="email" type="email"
class="form-control @error('email') is-invalid @enderror" name="register_email"
value="{{ old('email') }}" required autocomplete="email">
See the difference? I don't either. Except for autofocus, which is irrelevant in our case.
And you would think that it's ok, because those two forms POST to different URLs and perform different actions. But in case of failing validation, you get errors on both forms instead of one. For example, in case of invalid credentials when you login, you see this:
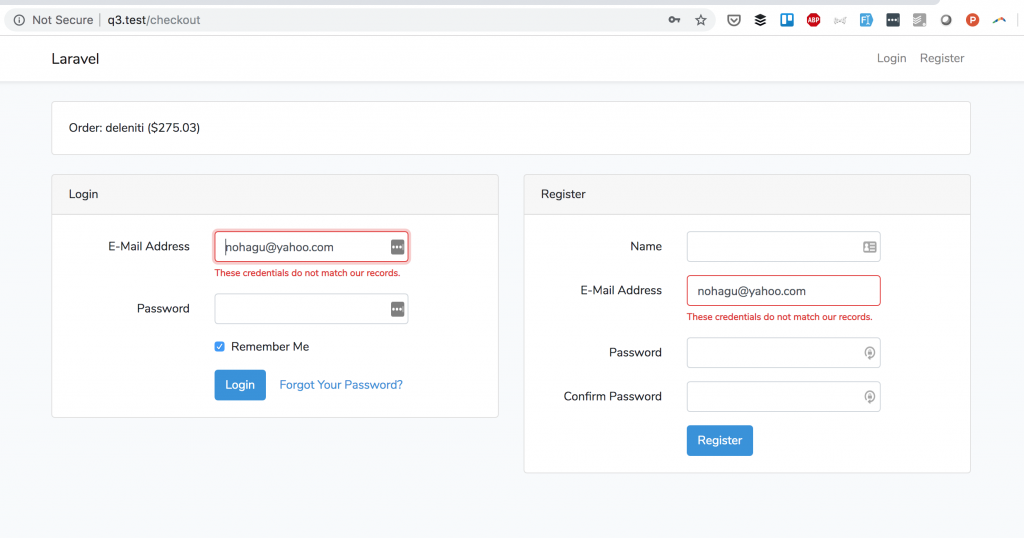
How to prevent it?
Step 1. Rename the fields in one of the form.
For this example, we will rename the fields in the registration part, adding "register_" prefix to them:
<input id="register_name" type="text"
class="form-control @error('register_name') is-invalid @enderror" name="register_name"
value="{{ old('register_name') }}" required autocomplete="name" autofocus>
@error('register_name')
<span class="invalid-feedback" role="alert">
<strong>{{ $message }}</strong>
</span>
@enderror
...
<input id="register_email" type="email"
class="form-control @error('register_email') is-invalid @enderror" name="register_email"
value="{{ old('register_email') }}" required autocomplete="email">
@error('register_email')
<span class="invalid-feedback" role="alert">
<strong>{{ $message }}</strong>
</span>
@enderror
...
<input id="register_password" type="password"
class="form-control @error('register_password') is-invalid @enderror"
name="register_password"
required autocomplete="new-password">
@error('register_password')
<span class="invalid-feedback" role="alert">
<strong>{{ $message }}</strong>
</span>
@enderror
...
<input id="password-confirm" type="password" class="form-control"
name="register_password_confirmation" required autocomplete="new-password">
Step 2. Override validator in RegisterController.
Here's the method validator() from the original app/Http/Controllers/Auth/RegisterController.php, that comes from the framework itself:
protected function validator(array $data)
{
return Validator::make($data, [
'name' => ['required', 'string', 'max:255'],
'email' => ['required', 'string', 'email', 'max:255', 'unique:users'],
'password' => ['required', 'string', 'min:8', 'confirmed'],
]);
}
So, we need to override that validator with our own, adding some rules to it. In fact, we just need to use the renamed fields from Step 1, and then assign their names properly. Here's the code:
protected function validator(array $data)
{
$validator = Validator::make($data, [
'register_name' => ['required', 'string', 'max:255'],
'register_email' => ['required', 'string', 'email', 'max:255', 'unique:users,email'],
'register_password' => ['required', 'string', 'min:8', 'confirmed'],
]);
$validator->setAttributeNames([
'register_name' => 'name',
'register_email' => 'email',
'register_password' => 'password',
]);
return $validator;
}
As you can see, we now use register_xxx fields instead of just xxx, but we also need to make sure they return validation errors with their "original" names, so for that we use less-known setAttributeNames() method. It's not officially mentioned in documentation, but you see another example of its use in this StackOverflow thread.
And that's it! Now, we have separate two forms on the same "checkout" page.
Bonus: E-shop Checkout Demo Project
As a part of this article, we actually created a typical checkout process mini-project, which is available on Github for you to see. Homepage contains list of products, you can buy each of them, and then session has your "cart" which you can checkout at any time, by clicking "Checkout" on top.
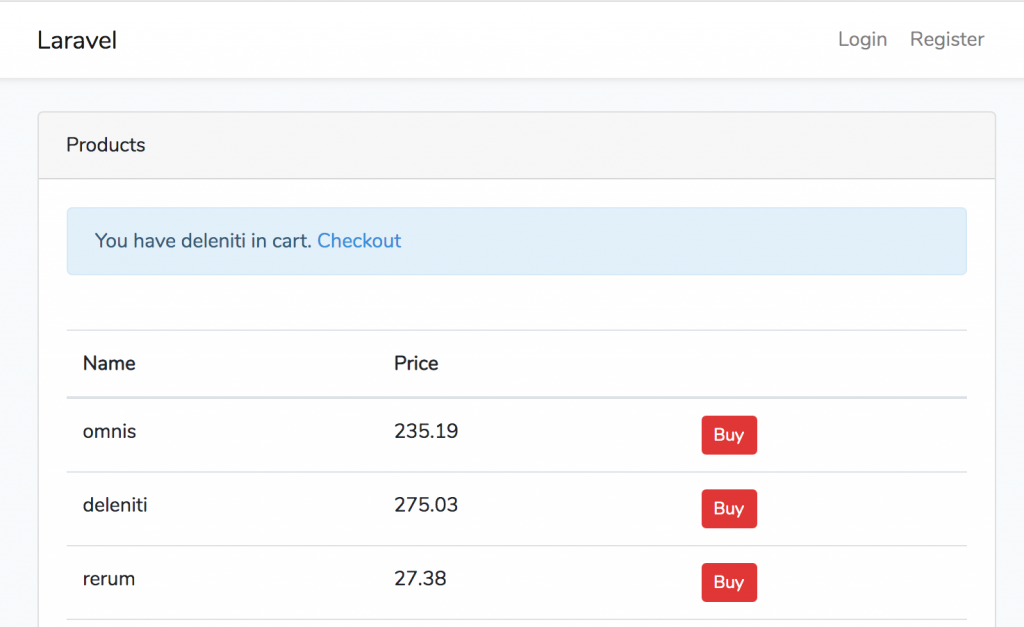
We didn't implement actual payment process, but I think it's still a useful example for Laravel juniors. Enjoy!
I`ve tried this. And the problem here is that func setAttributeNames() is working only for validator showing error, but not for column name in datatable. For specifying column name you should use rule like this: 'state' => 'exists:states,abbreviation'. https://laravel.com/docs/10.x/validation#specifying-a-custom-column-name