Another review in our series of Laravel projects. Confomo was created by an active Laravel community member and blogger Matt Stauffer, quoting him: "Built in 4 hours to help me track who I wanted to meet at Laracon 2014, and who I met there who I didn't know yet."
So, a tool with a real personal purpose, and built in hackaton-style way. But it's surprisingly good, although simple. Let's take a closer look.
Update: according to author Matt, version 2 of Confomo was largely built by Michael Dyrynda, credits go to him as well.
Other reviews in this series:
- FlarePoint: Laravel-based free CRM
- Invoice Ninja – Laravel-powered solution for better invoicing
- Attendize – event tickets selling system based on Laravel 5
- Ribbbon – project management system on Laravel 5.1 and Vue.js
- Faveo: impressive helpdesk system built on Laravel
- Confomo: Laravel-based website to meet Twitter friends at conferences
Installation
As a tool built by developer for (mostly) developers, installation is really easy and typical: git clone -> composer install -> .env file -> DB migrations. It all worked well, and I usually insert migrations screenshot in reviews - to evaluate the size of the database. In this case, it's really tiny.
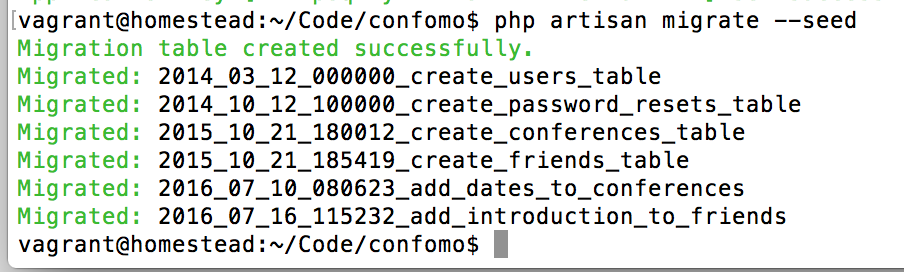
So not much to add to installation, the final result was this homepage.
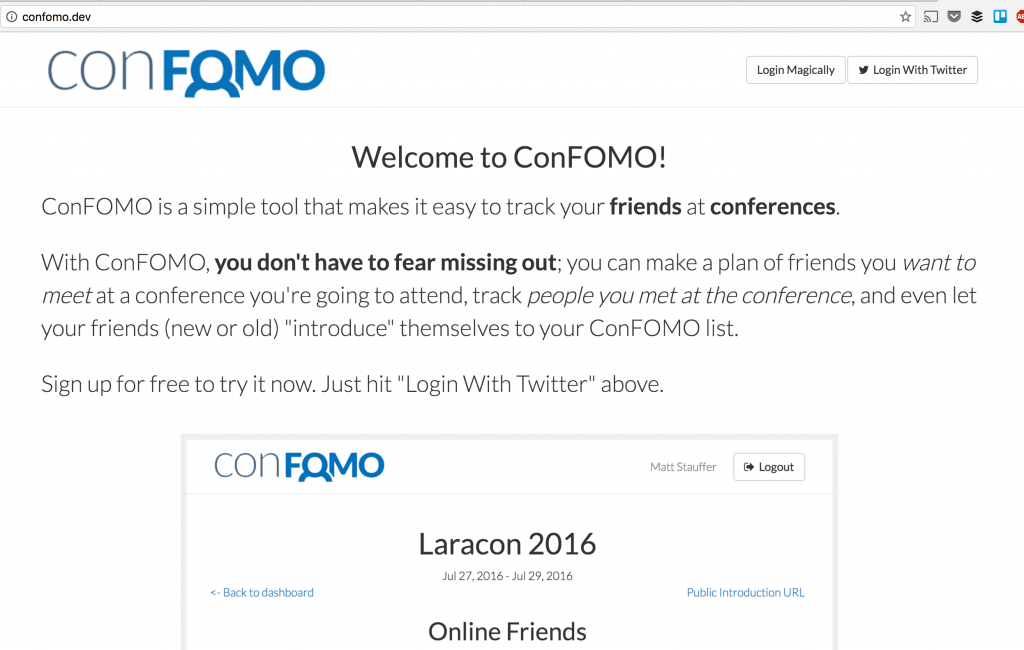
Fancy design? Who needs that?! That's the spirit of hackathon-type development for the purpose. Now, let's see how that invitation system actually works.
Usage
I love one tiny solution by the author - to insert a testing login. If you look at the top of the page, there are two ways to log in - "Login with Twitter" and "Login magically". What it does is logging in with a "fake" user that had been generated via seeds:
class DatabaseSeeder extends Seeder { private $faker; public function __construct(Faker\Generator $faker) { $this->faker = $faker; } /** * Run the database seeds. * * @return void */ public function run() { Model::unguard(); DB::table('users')->insert([ 'name' => $this->faker->name, 'twitter_id' => str_random(10), ]); Model::reguard(); } }
This "fake" login allows to test the system without integrating Twitter authorization (I would otherwise need to create a Twitter app etc.). Well done, Matt, thank you for saving my time.
Now, next thing we do is create our event - it's just a few fields.
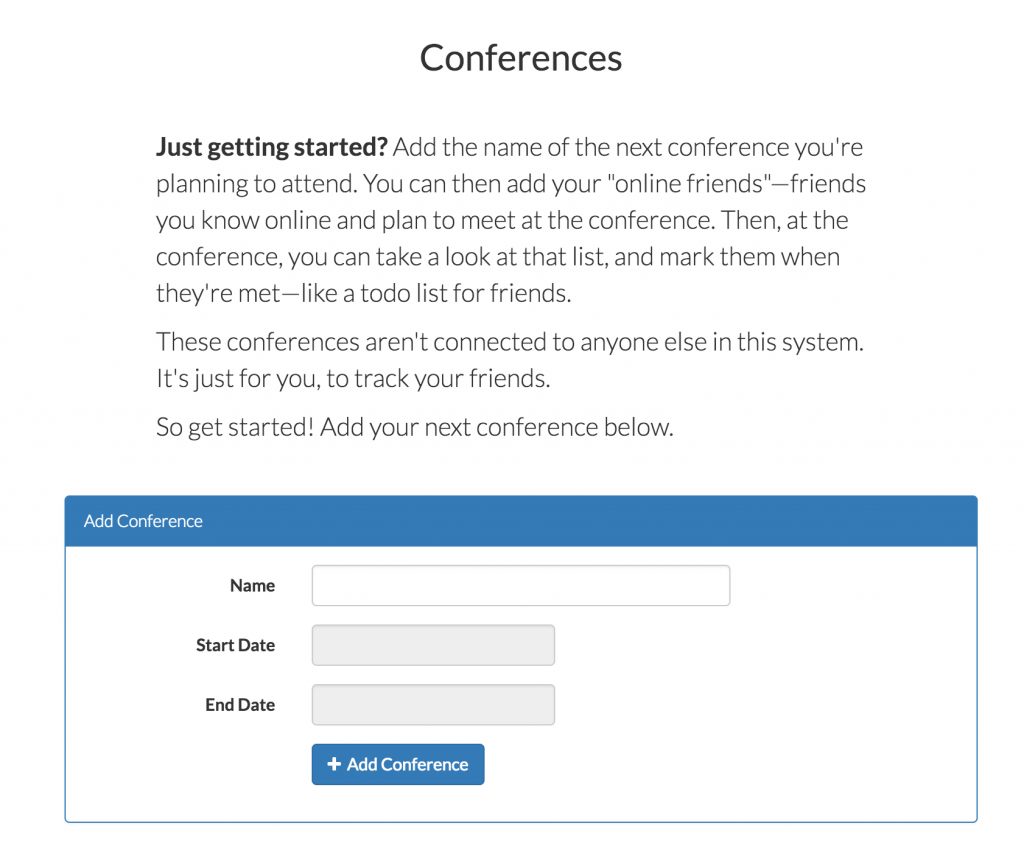
And then we can basically add Twitter usernames of people we know online but want to meet at the event. It's kind of a reminder, cause these days it's impossible to keep it all inside your head.
People are divided into two groups - those who you know, and those who you haven't met yet.
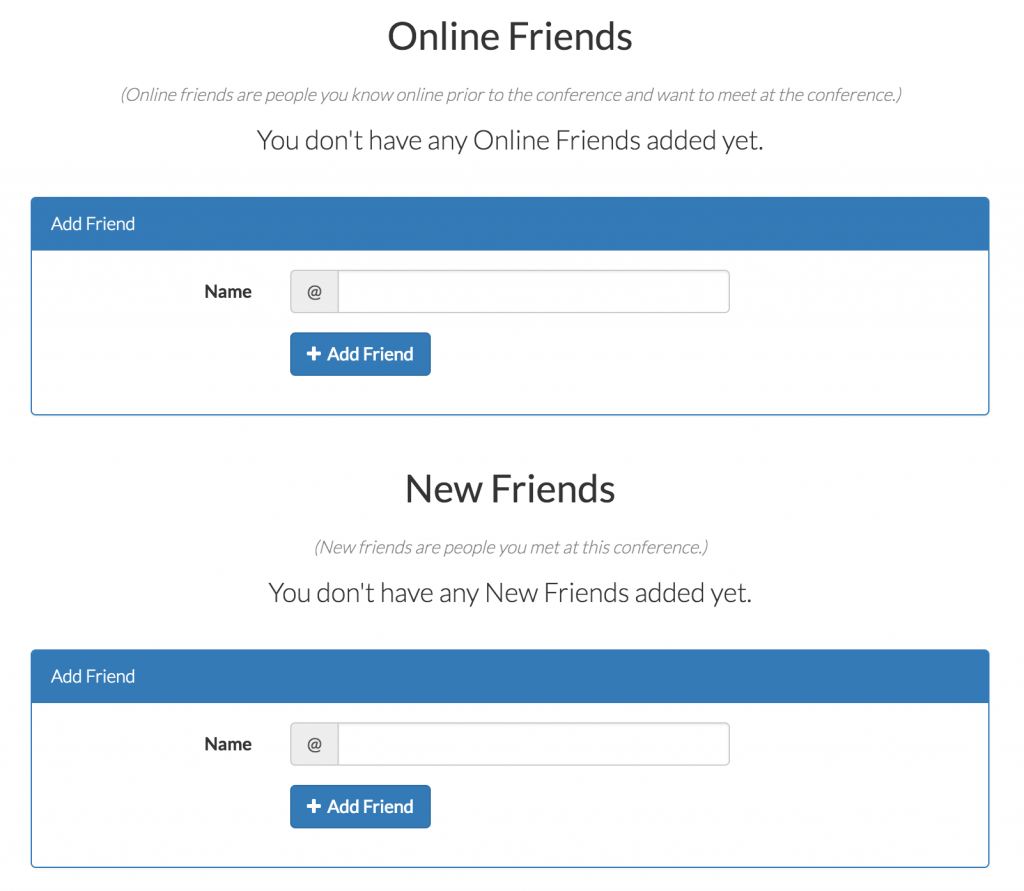
You just add their Twitter username and they appear in the list. Then you can delete them or mark as met - meaning that the goal has been achieved.

Finally, another nice feature is that you can invite others to be added to your list! For example, you want to tell the world that you're going to some event, and want others to get to meet you to say hello. Then Confomo gives you a personal invitation link for that particular event, it looks like this:
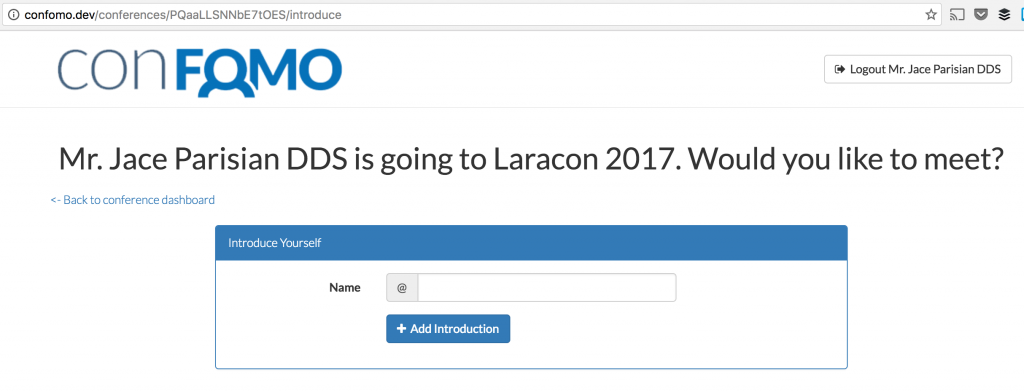
Quite impressive, given that it has been created in 4 hours, huh? Now, let's briefly look at the code.
Laravel code
As usual, I start code analysis from routes file - here we have version 5.1 so the file is at app/Http/routes.php. As expected, it's quite small:
Route::get('/', ['middleware' =--> 'guest', function () {
return view('home');
}]);
Route::group(['middleware' => 'auth'], function () {
Route::get('dashboard', function () {
return view('dashboard');
});
Route::get('conferences/{conference}', 'ConferencesController@show');
});
Route::group(['prefix' => 'api', 'namespace' => 'API', 'middleware' => 'auth'], function () {
Route::group(['prefix' => 'conferences'], function () {
Route::get('/', 'ConferencesController@index');
Route::post('/', 'ConferencesController@store');
Route::delete('{conference}', 'ConferencesController@delete');
Route::get('{conference}/new-friends', 'ConferenceNewFriendsController@index');
Route::post('{conference}/new-friends', 'ConferenceNewFriendsController@store');
Route::delete('{conference}/new-friends/{friend}', 'ConferenceNewFriendsController@delete');
Route::get('{conference}/online-friends', 'ConferenceOnlineFriendsController@index');
Route::get('{conference}/online-friends/{friend}', 'ConferenceOnlineFriendsController@show');
Route::post('{conference}/online-friends', 'ConferenceOnlineFriendsController@store');
Route::patch('{conference}/online-friends/{friend}', 'ConferenceOnlineFriendsController@update');
Route::delete('{conference}/online-friends/{friend}', 'ConferenceOnlineFriendsController@delete');
});
});
Route::get('local-login', 'Auth\AuthController@localLogin');
Route::get('auth', 'Auth\AuthController@authenticate');
Route::get('auth/callback', 'Auth\AuthController@handleTwitterCallback');
Route::get('auth/logout', 'Auth\AuthController@getLogout');
Route::get('avatar/{username}', 'AvatarController@show');
Route::get('conferences/{conferenceSlug}/introduce', 'ConferenceIntroductionController@index');
Route::post('api/conferences/{conferenceSlug}/introduction', 'ConferenceIntroductionController@store');
As you can see, there are 2 types of URLs - client-facing and API. That's kind of a new trend online - move all data management into an API and more functionality to the front-end.
Let's take a look at one of the Controllers now. Here is where we see beauty of Laravel "magic" - the code is so easy to read.
class ConferenceIntroductionController extends Controller { public function index($conferenceSlug) { return view('conferences.introduce') ->with('conference', Conference::where('slug', $conferenceSlug)->firstOrFail()); } public function store($conferenceSlug, Request $request) { $this->validate($request, ['username' => 'required']); return Conference::where('slug', $conferenceSlug) ->firstOrFail() ->makeIntroduction($request->input('username')); } }
Nested methods with error management like firstOrFail() - that's the spirit of using the framework to its fullest. Of course, what can you expect from the one and only Matt Stauffer?
In conclusion, Confomo is a nice little tool for event organizers and attendees to manage their friends list. And also a good example of Laravel 5 project.
Other reviews in this series:
- FlarePoint: Laravel-based free CRM
- Invoice Ninja – Laravel-powered solution for better invoicing
- Attendize – event tickets selling system based on Laravel 5
- Ribbbon – project management system on Laravel 5.1 and Vue.js
- Faveo: impressive helpdesk system built on Laravel
- Confomo: Laravel-based website to meet Twitter friends at conferences
No comments or questions yet...