public function posts() { return $this->hasMany(\App\Post::class); }Now, you need to realize that this wouldn't work:
$topics = Topic::with('posts')->orderBy('posts.created_at')->get();What we actually need to do - two things, actually: 1. Describe a separate relationship for the latest post in the topic:
public function latestPost() { return $this->hasOne(\App\Post::class)->latest(); }2. And then, in our controller, we can do this "magic":
$users = Topic::with('latestPost')->get()->sortByDesc('latestPost.created_at');
Let's test it out - here's our topics table.
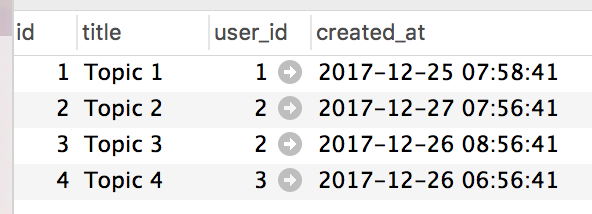
$users = User::with('latestPost')->get()->sortByDesc('latestPost.created_at'); foreach ($users as $user) { echo $user->id . ' - ' . $user->latestPost->title . ' (' . $user->latestPost->created_at . ')Result:
'; }
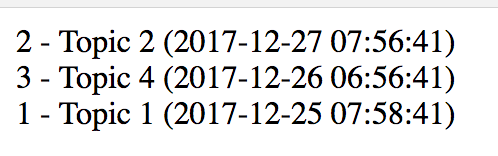
No comments or questions yet...