Today I want to share a small trick which I've found out only recently. Let's say you need to have a select dropdown field with number range from X to Y - for example, birth year from 1900 to 2015. How would you do it?
In general PHP, you can write HTML and for loop:
<select name="year">
<? for ($year=1900; $year <= 2015; $year++): ?>
<option value="<?=$year;?>"><?=$year;?></option>
<? endfor; ?>
</select>
Of course, in Laravel you have Form::select and you can pass array to it:
Controller:
$years = []; for ($year=1900; $year <= 2015; $year++) $years[$year] = $year;
View:
{!! Form::select('year', $years) !!}
But seems also quite long for such a simple operation, right? Luckily, there is a better and more elegant way - meet function selectRange().
{!! Form::selectRange('year', 1900, 2015) !!}
And that's it, here's the dropdown result:

As you probably understood, selectRange() has three parameters - field name, range start number and end number. Unfortunately, there's no parameter for step - so if you want to build a dropdown with numbers repeating every 2, every 5 or something - this function won't work for you, you have to build it manually.
Bonus: selectYear() and selectMonth()
Also there are two extra similar functions in illuminate/html package.
Actually, there's even shorter way to have a dropdown for years - with special function selectYear().
{!! Form::selectYear('year', 1900, 2015) !!}
It will produce the same result as the function selectRange() above.
And final tip - if you want to have dropdown of months - simple: selectMonth().
{!! Form::selectMonth('month') !!}
Here's how the result looks:
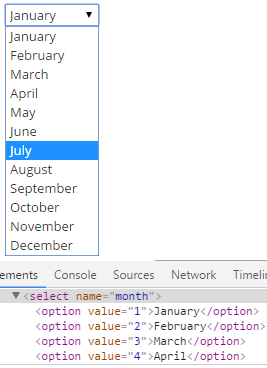
So there you go - a few sweet helpers to make your code prettier and shorter.
No comments or questions yet...