1. Laravel.io portal
URL: https://github.com/laravelio/portal Recently re-launched Laravel.io has its source published on Github. And Dries Vints has done quite a good job on writing tests there.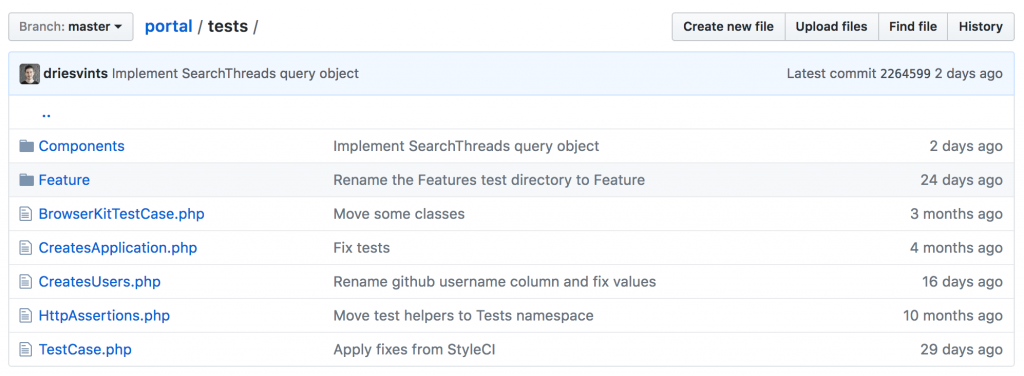
public function users_can_add_a_reply_to_a_thread() { factory(Thread::class)->create(['subject' => 'The first thread', 'slug' => 'the-first-thread']); $this->login(); $this->visit('/forum/the-first-thread') ->type('The first reply', 'body') ->press('Reply') ->see('The first thread') ->see('The first reply') ->see('Reply successfully added!'); }
public function we_can_create_a_reply() { $job = new CreateReply('Foo', '', $this->createUser(), factory(Thread::class)->create()); $this->assertInstanceOf(Reply::class, $job->handle()); }
Have you tried our tool to generate Laravel adminpanel without a line of code?
Go to QuickAdminPanel.com
2. Cachet - an open source status page system
URL: https://github.com/cachethq/Cachet Led by James Brooks and Graham Campbell, this project has a massive test suite. It's even hard to understand by looking at the surface.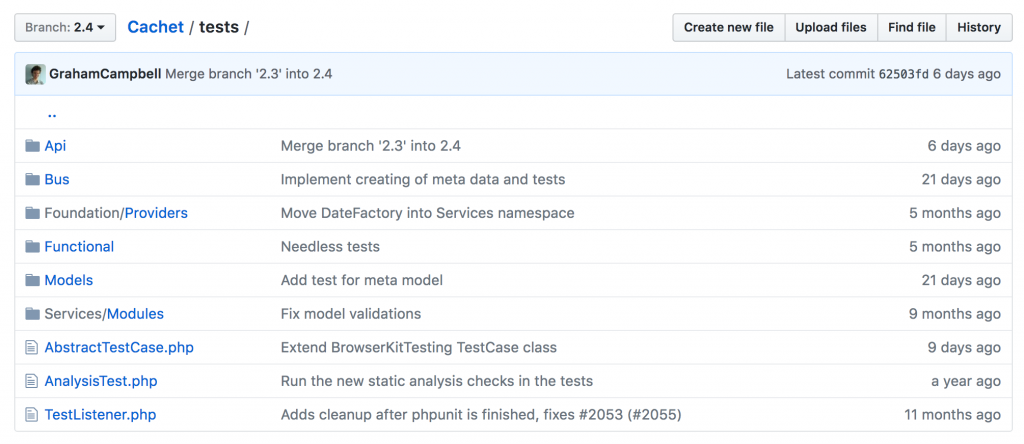
use AltThree\TestBench\ValidationTrait; use CachetHQ\Cachet\Models\Component; use CachetHQ\Tests\Cachet\AbstractTestCase; class ComponentTest extends AbstractTestCase { use ValidationTrait; public function testValidation() { $this->checkRules(new Component()); } }
3. October CMS
URL: https://github.com/octobercms/october No.1 Laravel-based CMS on the market, and it has pretty good testing suite.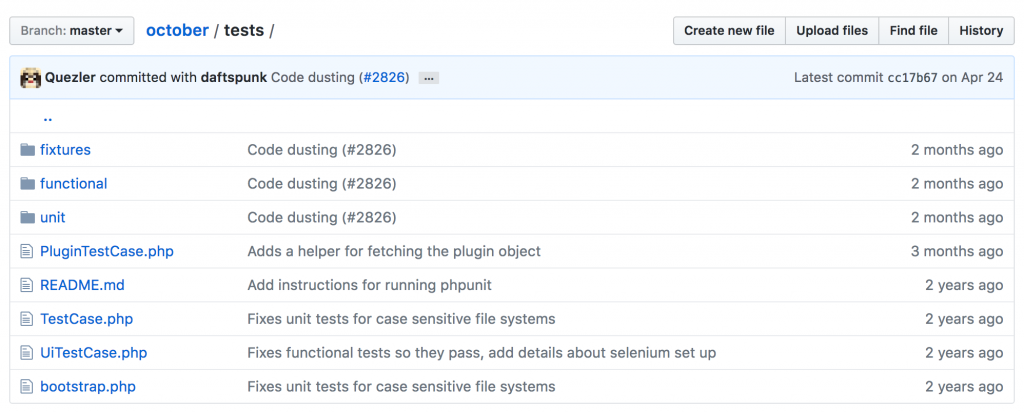
- Unit testing
- Functional testing
- Plugin testing
class ExportModelTest extends TestCase { // // Helpers // protected static function callProtectedMethod($object, $name, $params = []) { $className = get_class($object); $class = new ReflectionClass($className); $method = $class->getMethod($name); $method->setAccessible(true); return $method->invokeArgs($object, $params); } // // Tests // public function testEncodeArrayValue() { $model = new ExampleExportModel; $data = ['foo', 'bar']; $result = self::callProtectedMethod($model, 'encodeArrayValue', [$data]); $this->assertEquals('foo|bar', $result); $data = ['dps | heals | tank', 'paladin', 'berserker', 'gunner']; $result = self::callProtectedMethod($model, 'encodeArrayValue', [$data]); $this->assertEquals('dps \| heals \| tank|paladin|berserker|gunner', $result); $data = ['art direction', 'roman empire', 'sci-fi']; $result = self::callProtectedMethod($model, 'encodeArrayValue', [$data, '-']); $this->assertEquals('art direction-roman empire-sci\-fi', $result); } }
4. Orgmanager - Invite System for GitHub Organizations
URL: https://github.com/orgmanager/orgmanager This is quite a simple project by Miguel Piedrafita, Orgmanager's tests are also pretty simple and understandable. Also divided into Unit, Feature and API tests.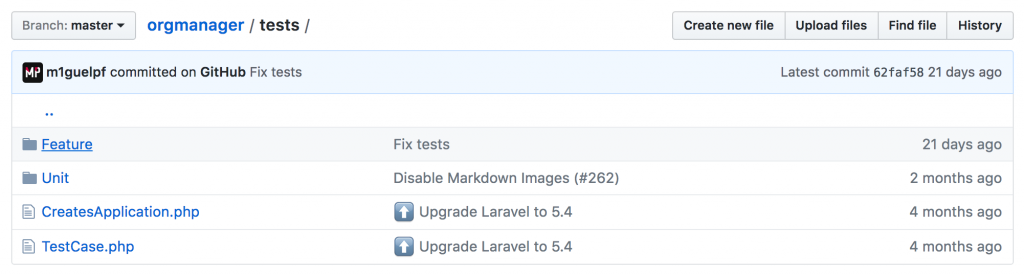
public function testJoinCommand() { $user = factory(User::class)->create(); $org = factory(Org::class)->create([ 'userid' => $user->id, ]); Github::shouldReceive('authenticate') ->once() ->with($org->user->token, null, 'http_token') ->andReturn(); Artisan::call('orgmanager:joinorg', [ 'org' => $org->id, 'username' => $user->github_username, ]); $this->assertEquals($user->github_username.' was invited to '.$org->name."\n", Artisan::output()); }
5. PHPMap
URL: https://github.com/PHPMap/phpmap Created and maintained by Florian Wartner.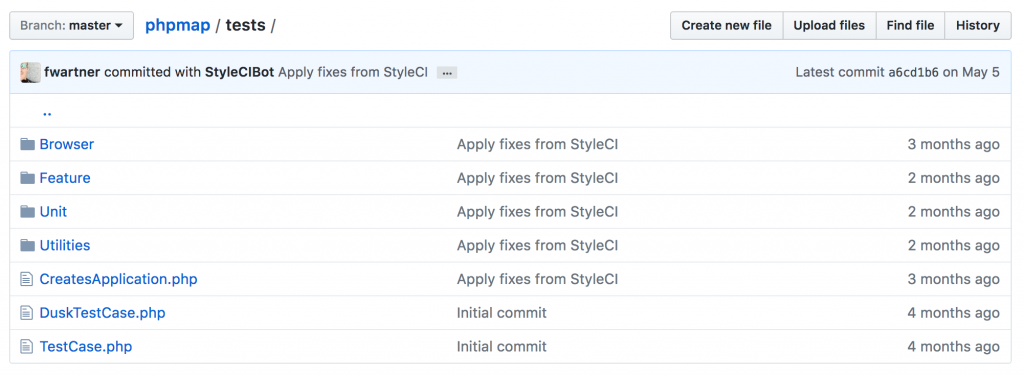
public function guests_can_not_favorite_anything() { $this->withExceptionHandling() ->post('forum/replies/1/favorites') ->assertRedirect('/login'); } public function an_authenticated_user_can_favorite_any_reply() { $this->signIn(); $reply = create('App\Models\Forum\Reply'); $this->post('forum/replies/'.$reply->id.'/forum/favorites'); $this->assertCount(1, $reply->favorites); }
public function testMap() { $this->browse(function ($browser) { $browser->visit('/map') ->assertSee('PHPMap'); }); }
6. Timegrid - Free, open-source, online appointments platform
URL: https://github.com/timegridio/timegrid The biggest contributor to Timegrid is Ariel Vallese, and he's done pretty awesome job with tests here.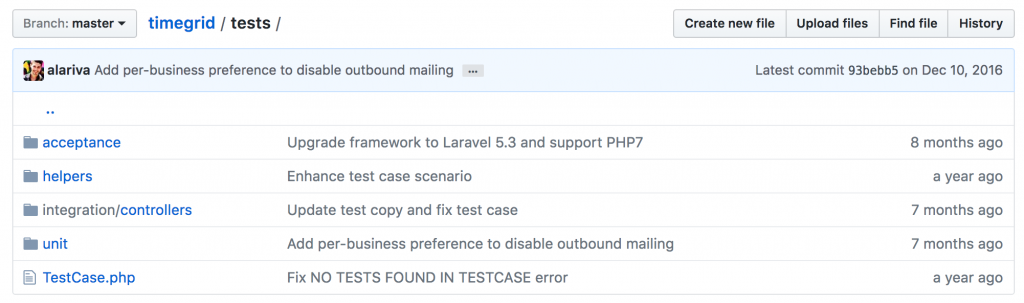
public function it_fits_for_consulting_scenario() { $this->arrangeScenario(); $this->the_business_publishes_a_consulting_service(); $this->the_business_publishes_vacancies(); $this->a_user_subscribes_to_business(); $this->the_user_queries_vacancies(); $this->it_provides_available_times_for_requested_service_date(); $this->the_user_takes_a_reservation(); $this->the_user_sees_the_reservation_ticket(); } public function the_business_publishes_a_consulting_service() { $this->service = $this->makeService([ 'name' => 'OnSite 4hs Support', 'duration' => 60 * 4, ]); $this->actingAs($this->owner); $this->call('POST', route('manager.business.service.store', $this->business), $this->service->toArray()); $this->assertCount(1, $this->business->fresh()->services); }
public function it_refreshes_rollbacks_and_seeds_the_database() { $database = env('DB_CONNECTION'); $this->assertNotNull($database); $exitCode = Artisan::call('migrate:refresh', ['--database' => $database]); $this->assertEquals(0, $exitCode); $exitCode = Artisan::call('migrate:rollback', ['--database' => $database]); $this->assertEquals(0, $exitCode); $exitCode = Artisan::call('migrate', ['--database' => $database]); $this->assertEquals(0, $exitCode); $exitCode = Artisan::call('db:seed', ['--database' => $database]); $this->assertEquals(0, $exitCode); }
Overall Conclusions
After looking at all of these different projects (and some I haven't mentioned here for various reasons), here are main takeaways I've made to myself about testing:- There's no choice between Unit "or" Feature testing - most of the projects have BOTH, or even more types of tests;
- Majority of projects use continuous integration (usually Travis) with test suite - otherwise, why bother writing tests?
- There are a lot of different ways to structure the tests - it totally depends on the project, there's no "silver bullet";
- Also a lot of ways to group internal testing functionality - helpers, abstract classes, seeding data etc. No rules, stick to what works for you.
- Migration to newer Laravel version may be pretty painful - for example, 5.3 tests look different from 5.4 version. So you need to think ahead about updates.
- Same thought from different angle - as your application grows, you would have to come back and change/add tests. In these projects I "feel" some legacy code, just because of some tests not being used anymore.
No comments or questions yet...